Struct libadwaita::AboutWindow
source · pub struct AboutWindow { /* private fields */ }
v1_2
only.Expand description
A window showing information about the application.
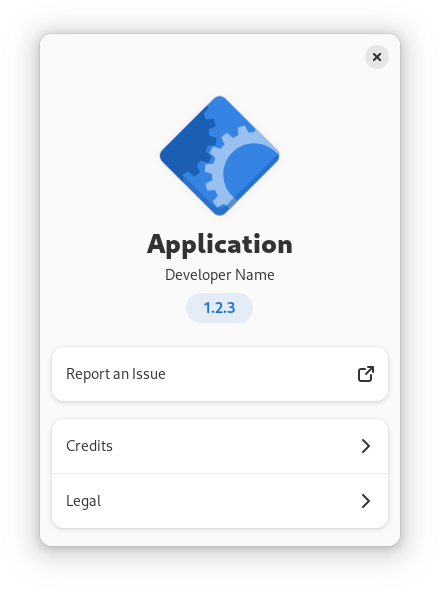
An about window is typically opened when the user activates the About …
item in the application’s primary menu. All parts of the window are optional.
§Main page
AboutWindow
prominently displays the application’s icon, name, developer
name and version. They can be set with the application-icon
,
application-name
,
developer-name
and version
respectively.
§What’s New
AboutWindow
provides a way for applications to display their release
notes, set with the release-notes
property.
Release notes are formatted the same way as AppStream descriptions.
The supported formatting options are:
- Paragraph (
<p>
) - Ordered list (
<ol>
), with list items (<li>
) - Unordered list (
<ul>
), with list items (<li>
)
Within paragraphs and list items, emphasis (<em>
) and inline code
(<code>
) text styles are supported. The emphasis is rendered in italic,
while inline code is shown in a monospaced font.
Any text outside paragraphs or list items is ignored.
Nested lists are not supported.
Only one version can be shown at a time. By default, the displayed version
number matches version
. Use
release-notes-version
to override it.
§Details
The Details page displays the application comments and links.
The comments can be set with the comments
property.
Unlike [comments
][struct@crate::Gtk::AboutDialog#comments], this string can be long and
detailed. It can also contain links and Pango markup.
To set the application website, use website
.
To add extra links below the website, use add_link()
.
If the Details page doesn’t have any other content besides website, the website will be displayed on the main page instead.
§Troubleshooting
AboutWindow
displays the following two links on the main page:
- Support Questions, set with the
support-url
property, - Report an Issue, set with the
issue-url
property.
Additionally, applications can provide debugging information. It will be
shown separately on the Troubleshooting page. Use the
debug-info
property to specify it.
It’s intended to be attached to issue reports when reporting issues against the application. As such, it cannot contain markup or links.
AboutWindow
provides a quick way to save debug information to a file.
When saving, debug-info-filename
would be used as
the suggested filename.
§Credits and Acknowledgements
The Credits page has the following default sections:
- Developers, set with the
developers
property, - Designers, set with the
designers
property, - Artists, set with the
artists
property, - Documenters, set with the
documenters
property, - Translators, set with the
translator-credits
property.
When setting translator credits, use the strings "translator-credits"
or
"translator_credits"
and mark them as translatable.
The default sections that don’t contain any names won’t be displayed.
The Credits page can also contain an arbitrary number of extra sections below
the default ones. Use add_credit_section()
to add them.
The Acknowledgements page can be used to acknowledge additional people and
organizations for their non-development contributions. Use
add_acknowledgement_section()
to add sections to it. For
example, it can be used to list backers in a crowdfunded project or to give
special thanks.
Each of the people or organizations can have an email address or a website
specified. To add a email address, use a string like
Edgar Allan Poe <edgar@poe.com>
. To specify a website with a title, use a
string like The GNOME Project https://www.gnome.org
:
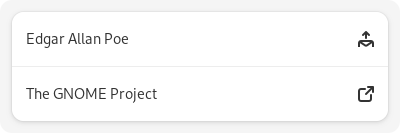
§Legal
The Legal page displays the copyright and licensing information for the application and other modules.
The copyright string is set with the copyright
property and should be a short string of one or two lines, for example:
© 2022 Example
.
Licensing information can be quickly set from a list of known licenses with
the license-type
property. If the application’s
license is not in the list, license
can be used
instead.
To add information about other modules, such as application dependencies or
data, use add_legal_section()
.
§Constructing
To make constructing an AboutWindow
as convenient as possible, you can
use the function show_about_window()
which constructs and shows a
window.
⚠️ The following code is in c ⚠️
static void
show_about (GtkApplication *app)
{
const char *developers[] = {
"Angela Avery",
NULL
};
const char *designers[] = {
"GNOME Design Team",
NULL
};
adw_show_about_window (gtk_application_get_active_window (app),
"application-name", _("Example"),
"application-icon", "org.example.App",
"version", "1.2.3",
"copyright", "© 2022 Angela Avery",
"issue-url", "https://gitlab.gnome.org/example/example/-/issues/new",
"license-type", GTK_LICENSE_GPL_3_0,
"developers", developers,
"designers", designers,
"translator-credits", _("translator-credits"),
NULL);
}
§CSS nodes
AboutWindow
has a main CSS node with the name window
and the
style class .about
.
§Properties
§application-icon
The name of the application icon.
The icon is displayed at the top of the main page.
Readable | Writeable
§application-name
The name of the application.
The name is displayed at the top of the main page.
Readable | Writeable
§artists
The list of artists of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
developers
designers
documenters
translator-credits
AboutWindow::add_credit_section()
AboutWindow::add_acknowledgement_section()
Readable | Writeable
§comments
The comments about the application.
Comments will be shown on the Details page, above links.
Unlike [comments
][struct@crate::Gtk::AboutDialog#comments], this string can be long and
detailed. It can also contain links and Pango markup.
Readable | Writeable
§copyright
The copyright information.
This should be a short string of one or two lines, for example:
© 2022 Example
.
The copyright information will be displayed on the Legal page, above the application license.
AboutWindow::add_legal_section()
can be used to add copyright
information for the application dependencies or other components.
Readable | Writeable
§debug-info
The debug information.
Debug information will be shown on the Troubleshooting page. It’s intended to be attached to issue reports when reporting issues against the application.
AboutWindow
provides a quick way to save debug information to a file.
When saving, debug-info-filename
would be used as
the suggested filename.
Debug information cannot contain markup or links.
Readable | Writeable
§debug-info-filename
The debug information filename.
It will be used as the suggested filename when saving debug information to a file.
See debug-info
.
Readable | Writeable
§designers
The list of designers of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
developers
artists
documenters
translator-credits
AboutWindow::add_credit_section()
AboutWindow::add_acknowledgement_section()
Readable | Writeable
§developer-name
The developer name.
The developer name is displayed on the main page, under the application name.
If the application is developed by multiple people, the developer name can
be set to values like “AppName team”, “AppName developers” or
“The AppName project”, and the individual contributors can be listed on the
Credits page, with developers
and related
properties.
Readable | Writeable
§developers
The list of developers of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
designers
artists
documenters
translator-credits
AboutWindow::add_credit_section()
AboutWindow::add_acknowledgement_section()
Readable | Writeable
§documenters
The list of documenters of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
developers
designers
artists
translator-credits
AboutWindow::add_credit_section()
AboutWindow::add_acknowledgement_section()
Readable | Writeable
§issue-url
The URL for the application’s issue tracker.
The issue tracker link is displayed on the main page.
Readable | Writeable
§license
The license text.
This can be used to set a custom text for the license if it can’t be set
via license-type
.
When set, license-type
will be set to
GTK_LICENSE_CUSTOM
.
The license text will be displayed on the Legal page, below the copyright information.
License text can contain Pango markup and links.
AboutWindow::add_legal_section()
can be used to add license
information for the application dependencies or other components.
Readable | Writeable
§license-type
The license type.
Allows to set the application’s license froma list of known licenses.
If the application’s license is not in the list,
license
can be used instead. The license type will
be automatically set to GTK_LICENSE_CUSTOM
in that case.
If set to GTK_LICENSE_UNKNOWN
, no information will be displayed.
If the license type is different from GTK_LICENSE_CUSTOM
.
license
will be cleared out.
The license description will be displayed on the Legal page, below the copyright information.
AboutWindow::add_legal_section()
can be used to add license
information for the application dependencies or other components.
Readable | Writeable
§release-notes
The release notes of the application.
Release notes are displayed on the the What’s New page.
Release notes are formatted the same way as AppStream descriptions.
The supported formatting options are:
- Paragraph (
<p>
) - Ordered list (
<ol>
), with list items (<li>
) - Unordered list (
<ul>
), with list items (<li>
)
Within paragraphs and list items, emphasis (<em>
) and inline code
(<code>
) text styles are supported. The emphasis is rendered in italic,
while inline code is shown in a monospaced font.
Any text outside paragraphs or list items is ignored.
Nested lists are not supported.
AboutWindow
displays the version above the release notes. If set, the
release-notes-version
of the property will be used
as the version; otherwise, version
is used.
Readable | Writeable
§release-notes-version
The version described by the application’s release notes.
The release notes version is displayed on the What’s New page, above the release notes.
If not set, version
will be used instead.
For example, an application with the current version 2.0.2 might want to keep the release notes from 2.0.0, and set the release notes version accordingly.
See release-notes
.
Readable | Writeable
§support-url
The URL of the application’s support page.
The support page link is displayed on the main page.
Readable | Writeable
§translator-credits
The translator credits string.
It will be displayed on the Credits page.
This string should be "translator-credits"
or "translator_credits"
and
should be marked as translatable.
The string may contain email addresses and URLs, see the introduction for more details.
See also:
developers
designers
artists
documenters
AboutWindow::add_credit_section()
AboutWindow::add_acknowledgement_section()
Readable | Writeable
§version
The version of the application.
The version is displayed on the main page.
If release-notes-version
is not set, the version
will also be displayed above the release notes on the What’s New page.
Readable | Writeable
§website
The URL of the application’s website.
Website is displayed on the Details page, below comments, or on the main page if the Details page doesn’t have any other content.
Applications can add other links below, see AboutWindow::add_link()
.
Readable | Writeable
Window
§content
The content widget.
This property should always be used instead of child
.
Readable | Writeable
§current-breakpoint
The current breakpoint.
Readable
§dialogs
The open dialogs.
Readable
§visible-dialog
The currently visible dialog
Readable
Window
§application
The gtk::Application
associated with the window.
The application will be kept alive for at least as long as it has any windows associated with it (see g_application_hold() for a way to keep it alive without windows).
Normally, the connection between the application and the window
will remain until the window is destroyed, but you can explicitly
remove it by setting the :application property to None
.
Readable | Writeable
§child
The child widget.
Readable | Writeable
§decorated
Whether the window should have a frame (also known as decorations).
Readable | Writeable
§default-height
The default height of the window.
Readable | Writeable
§default-widget
The default widget.
Readable | Writeable
§default-width
The default width of the window.
Readable | Writeable
§deletable
Whether the window frame should have a close button.
Readable | Writeable
§destroy-with-parent
If this window should be destroyed when the parent is destroyed.
Readable | Writeable
§display
The display that will display this window.
Readable | Writeable
§focus-visible
Whether ‘focus rectangles’ are currently visible in this window.
This property is maintained by GTK based on user input and should not be set by applications.
Readable | Writeable
§focus-widget
The focus widget.
Readable | Writeable
§fullscreened
Whether the window is fullscreen.
Setting this property is the equivalent of calling
[GtkWindowExtManual::fullscreen()
][crate::gtk::prelude::GtkWindowExtManual::fullscreen()] or [GtkWindowExtManual::unfullscreen()
][crate::gtk::prelude::GtkWindowExtManual::unfullscreen()];
either operation is asynchronous, which means you will need to
connect to the ::notify signal in order to know whether the
operation was successful.
Readable | Writeable | Construct
§handle-menubar-accel
Whether the window frame should handle F10 for activating menubars.
Readable | Writeable
§hide-on-close
If this window should be hidden when the users clicks the close button.
Readable | Writeable
§icon-name
Specifies the name of the themed icon to use as the window icon.
See Gtk::IconTheme
for more details.
Readable | Writeable
§is-active
Whether the toplevel is the currently active window.
Readable
§maximized
Whether the window is maximized.
Setting this property is the equivalent of calling
[GtkWindowExtManual::maximize()
][crate::gtk::prelude::GtkWindowExtManual::maximize()] or [GtkWindowExtManual::unmaximize()
][crate::gtk::prelude::GtkWindowExtManual::unmaximize()];
either operation is asynchronous, which means you will need to
connect to the ::notify signal in order to know whether the
operation was successful.
Readable | Writeable | Construct
§mnemonics-visible
Whether mnemonics are currently visible in this window.
This property is maintained by GTK based on user input, and should not be set by applications.
Readable | Writeable
§modal
If true
, the window is modal.
Readable | Writeable
§resizable
If true
, users can resize the window.
Readable | Writeable
§startup-id
A write-only property for setting window’s startup notification identifier.
Writeable
§suspended
Whether the window is suspended.
See [GtkWindowExtManual::is_suspended()
][crate::gtk::prelude::GtkWindowExtManual::is_suspended()] for details about what suspended means.
Readable
§title
The title of the window.
Readable | Writeable
§titlebar
The titlebar widget.
Readable | Writeable
§transient-for
The transient parent of the window.
Readable | Writeable | Construct
Widget
§can-focus
Whether the widget or any of its descendents can accept the input focus.
This property is meant to be set by widget implementations, typically in their instance init function.
Readable | Writeable
§can-target
Whether the widget can receive pointer events.
Readable | Writeable
§css-classes
A list of css classes applied to this widget.
Readable | Writeable
§css-name
The name of this widget in the CSS tree.
This property is meant to be set by widget implementations, typically in their instance init function.
Readable | Writeable | Construct Only
§cursor
The cursor used by @widget.
Readable | Writeable
§focus-on-click
Whether the widget should grab focus when it is clicked with the mouse.
This property is only relevant for widgets that can take focus.
Readable | Writeable
§focusable
Whether this widget itself will accept the input focus.
Readable | Writeable
§halign
How to distribute horizontal space if widget gets extra space.
Readable | Writeable
§has-default
Whether the widget is the default widget.
Readable
§has-focus
Whether the widget has the input focus.
Readable
§has-tooltip
Enables or disables the emission of the ::query-tooltip signal on @widget.
A value of true
indicates that @widget can have a tooltip, in this case
the widget will be queried using query-tooltip
to
determine whether it will provide a tooltip or not.
Readable | Writeable
§height-request
Override for height request of the widget.
If this is -1, the natural request will be used.
Readable | Writeable
§hexpand
Whether to expand horizontally.
Readable | Writeable
§hexpand-set
Whether to use the hexpand
property.
Readable | Writeable
§layout-manager
The gtk::LayoutManager
instance to use to compute the preferred size
of the widget, and allocate its children.
This property is meant to be set by widget implementations, typically in their instance init function.
Readable | Writeable
§margin-bottom
Margin on bottom side of widget.
This property adds margin outside of the widget’s normal size
request, the margin will be added in addition to the size from
[WidgetExtManual::set_size_request()
][crate::gtk::prelude::WidgetExtManual::set_size_request()] for example.
Readable | Writeable
§margin-end
Margin on end of widget, horizontally.
This property supports left-to-right and right-to-left text directions.
This property adds margin outside of the widget’s normal size
request, the margin will be added in addition to the size from
[WidgetExtManual::set_size_request()
][crate::gtk::prelude::WidgetExtManual::set_size_request()] for example.
Readable | Writeable
§margin-start
Margin on start of widget, horizontally.
This property supports left-to-right and right-to-left text directions.
This property adds margin outside of the widget’s normal size
request, the margin will be added in addition to the size from
[WidgetExtManual::set_size_request()
][crate::gtk::prelude::WidgetExtManual::set_size_request()] for example.
Readable | Writeable
§margin-top
Margin on top side of widget.
This property adds margin outside of the widget’s normal size
request, the margin will be added in addition to the size from
[WidgetExtManual::set_size_request()
][crate::gtk::prelude::WidgetExtManual::set_size_request()] for example.
Readable | Writeable
§name
The name of the widget.
Readable | Writeable
§opacity
The requested opacity of the widget.
Readable | Writeable
§overflow
How content outside the widget’s content area is treated.
This property is meant to be set by widget implementations, typically in their instance init function.
Readable | Writeable
§parent
The parent widget of this widget.
Readable
§receives-default
Whether the widget will receive the default action when it is focused.
Readable | Writeable
§root
The gtk::Root
widget of the widget tree containing this widget.
This will be None
if the widget is not contained in a root widget.
Readable
§scale-factor
The scale factor of the widget.
Readable
§sensitive
Whether the widget responds to input.
Readable | Writeable
§tooltip-markup
Sets the text of tooltip to be the given string, which is marked up with Pango markup.
Also see Gtk::Tooltip::set_markup()
.
This is a convenience property which will take care of getting the
tooltip shown if the given string is not None
:
has-tooltip
will automatically be set to true
and there will be taken care of query-tooltip
in
the default signal handler.
Note that if both tooltip-text
and
tooltip-markup
are set, the last one wins.
Readable | Writeable
§tooltip-text
Sets the text of tooltip to be the given string.
Also see Gtk::Tooltip::set_text()
.
This is a convenience property which will take care of getting the
tooltip shown if the given string is not None
:
has-tooltip
will automatically be set to true
and there will be taken care of query-tooltip
in
the default signal handler.
Note that if both tooltip-text
and
tooltip-markup
are set, the last one wins.
Readable | Writeable
§valign
How to distribute vertical space if widget gets extra space.
Readable | Writeable
§vexpand
Whether to expand vertically.
Readable | Writeable
§vexpand-set
Whether to use the vexpand
property.
Readable | Writeable
§visible
Whether the widget is visible.
Readable | Writeable
§width-request
Override for width request of the widget.
If this is -1, the natural request will be used.
Readable | Writeable
Accessible
§accessible-role
The accessible role of the given gtk::Accessible
implementation.
The accessible role cannot be changed once set.
Readable | Writeable
§Signals
§activate-link
Emitted when a URL is activated.
Applications may connect to it to override the default behavior, which is
to call show_uri()
.
Window
§activate-default
Emitted when the user activates the default widget of @window.
This is a keybinding signal.
Action
§activate-focus
Emitted when the user activates the currently focused widget of @window.
This is a keybinding signal.
Action
§close-request
Emitted when the user clicks on the close button of the window.
§enable-debugging
Emitted when the user enables or disables interactive debugging.
When @toggle is true
, interactive debugging is toggled on or off,
when it is false
, the debugger will be pointed at the widget
under the pointer.
This is a keybinding signal.
The default bindings for this signal are Ctrl-Shift-I and Ctrl-Shift-D.
Action
§keys-changed
emitted when the set of accelerators or mnemonics that are associated with @window changes.
Widget
§destroy
Signals that all holders of a reference to the widget should release the reference that they hold.
May result in finalization of the widget if all references are released.
This signal is not suitable for saving widget state.
§direction-changed
Emitted when the text direction of a widget changes.
§hide
Emitted when @widget is hidden.
§keynav-failed
Emitted if keyboard navigation fails.
See [WidgetExtManual::keynav_failed()
][crate::gtk::prelude::WidgetExtManual::keynav_failed()] for details.
§map
Emitted when @widget is going to be mapped.
A widget is mapped when the widget is visible (which is controlled with
visible
) and all its parents up to the toplevel widget
are also visible.
The ::map signal can be used to determine whether a widget will be drawn,
for instance it can resume an animation that was stopped during the
emission of unmap
.
§mnemonic-activate
Emitted when a widget is activated via a mnemonic.
The default handler for this signal activates @widget if @group_cycling
is false
, or just makes @widget grab focus if @group_cycling is true
.
§move-focus
Emitted when the focus is moved.
The ::move-focus signal is a keybinding signal.
The default bindings for this signal are Tab to move forward, and Shift+Tab to move backward.
Action
§query-tooltip
Emitted when the widget’s tooltip is about to be shown.
This happens when the has-tooltip
property
is true
and the hover timeout has expired with the cursor hovering
“above” @widget; or emitted when @widget got focus in keyboard mode.
Using the given coordinates, the signal handler should determine
whether a tooltip should be shown for @widget. If this is the case
true
should be returned, false
otherwise. Note that if
@keyboard_mode is true
, the values of @x and @y are undefined and
should not be used.
The signal handler is free to manipulate @tooltip with the therefore destined function calls.
§realize
Emitted when @widget is associated with a GdkSurface
.
This means that [WidgetExtManual::realize()
][crate::gtk::prelude::WidgetExtManual::realize()] has been called
or the widget has been mapped (that is, it is going to be drawn).
§show
Emitted when @widget is shown.
§state-flags-changed
Emitted when the widget state changes.
See [WidgetExtManual::state_flags()
][crate::gtk::prelude::WidgetExtManual::state_flags()].
§unmap
Emitted when @widget is going to be unmapped.
A widget is unmapped when either it or any of its parents up to the toplevel widget have been set as hidden.
As ::unmap indicates that a widget will not be shown any longer, it can be used to, for example, stop an animation on the widget.
§unrealize
Emitted when the GdkSurface
associated with @widget is destroyed.
This means that [WidgetExtManual::unrealize()
][crate::gtk::prelude::WidgetExtManual::unrealize()] has been called
or the widget has been unmapped (that is, it is going to be hidden).
§Implements
AdwWindowExt
, gtk::prelude::GtkWindowExt
, gtk::prelude::WidgetExt
, [trait@glib::ObjectExt
], gtk::prelude::AccessibleExt
, gtk::prelude::BuildableExt
, gtk::prelude::ConstraintTargetExt
, gtk::prelude::NativeExt
, gtk::prelude::RootExt
, gtk::prelude::ShortcutManagerExt
Implementations§
source§impl AboutWindow
impl AboutWindow
sourcepub fn new() -> AboutWindow
pub fn new() -> AboutWindow
sourcepub fn from_appdata(
resource_path: &str,
release_notes_version: Option<&str>
) -> AboutWindow
Available on Linux and crate feature v1_4
only.
pub fn from_appdata( resource_path: &str, release_notes_version: Option<&str> ) -> AboutWindow
v1_4
only.Creates a new AboutWindow
using AppStream metadata.
This automatically sets the following properties with the following AppStream values:
application-icon
is set from the<id>
application-name
is set from the<name>
developer-name
is set from the<developer_name>
version
is set from the version of the latest releasewebsite
is set from the<url type="homepage">
support-url
is set from the<url type="help">
issue-url
is set from the<url type="bugtracker">
license-type
is set from the<project_license>
If the license type retrieved from AppStream is not listed ingtk::License
, it will be set toGTK_LICENCE_CUSTOM
.
If @release_notes_version is not NULL
,
release-notes-version
is set to match it, while
release-notes
is set from the AppStream release
description for that version.
§resource_path
The resource to use
§release_notes_version
The version to retrieve release notes for
§Returns
the newly created AboutWindow
sourcepub fn builder() -> AboutWindowBuilder
pub fn builder() -> AboutWindowBuilder
Creates a new builder-pattern struct instance to construct AboutWindow
objects.
This method returns an instance of AboutWindowBuilder
which can be used to create AboutWindow
objects.
sourcepub fn add_acknowledgement_section(&self, name: Option<&str>, people: &[&str])
pub fn add_acknowledgement_section(&self, name: Option<&str>, people: &[&str])
Adds a section to the Acknowledgements page.
This can be used to acknowledge additional people and organizations for their non-development contributions - for example, backers in a crowdfunded project.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
§name
the section name
§people
the list of names
sourcepub fn add_credit_section(&self, name: Option<&str>, people: &[&str])
pub fn add_credit_section(&self, name: Option<&str>, people: &[&str])
sourcepub fn add_legal_section(
&self,
title: &str,
copyright: Option<&str>,
license_type: License,
license: Option<&str>
)
pub fn add_legal_section( &self, title: &str, copyright: Option<&str>, license_type: License, license: Option<&str> )
Adds an extra section to the Legal page.
Extra sections will be displayed below the application’s own information.
The parameters @copyright, @license_type and @license will be used to present
the it the same way as copyright
,
license-type
and license
are
for the application’s own information.
See those properties for more details.
This can be useful to attribute the application dependencies or data.
Examples:
⚠️ The following code is in c ⚠️
adw_about_window_add_legal_section (ADW_ABOUT_WINDOW (about),
_("Copyright and a known license"),
"© 2022 Example",
GTK_LICENSE_LGPL_2_1,
NULL);
adw_about_window_add_legal_section (ADW_ABOUT_WINDOW (about),
_("Copyright and custom license"),
"© 2022 Example",
GTK_LICENSE_CUSTOM,
"Custom license text");
adw_about_window_add_legal_section (ADW_ABOUT_WINDOW (about),
_("Copyright only"),
"© 2022 Example",
GTK_LICENSE_UNKNOWN,
NULL);
adw_about_window_add_legal_section (ADW_ABOUT_WINDOW (about),
_("Custom license only"),
NULL,
GTK_LICENSE_CUSTOM,
"Something completely custom here.");
§title
the name of the section
§copyright
a copyright string
§license_type
the type of license
§license
custom license information
sourcepub fn application_icon(&self) -> GString
pub fn application_icon(&self) -> GString
sourcepub fn application_name(&self) -> GString
pub fn application_name(&self) -> GString
sourcepub fn debug_info(&self) -> GString
pub fn debug_info(&self) -> GString
sourcepub fn debug_info_filename(&self) -> GString
pub fn debug_info_filename(&self) -> GString
sourcepub fn developer_name(&self) -> GString
pub fn developer_name(&self) -> GString
sourcepub fn developers(&self) -> Vec<GString>
pub fn developers(&self) -> Vec<GString>
sourcepub fn documenters(&self) -> Vec<GString>
pub fn documenters(&self) -> Vec<GString>
sourcepub fn license_type(&self) -> License
pub fn license_type(&self) -> License
sourcepub fn release_notes(&self) -> GString
pub fn release_notes(&self) -> GString
sourcepub fn release_notes_version(&self) -> GString
pub fn release_notes_version(&self) -> GString
sourcepub fn support_url(&self) -> GString
pub fn support_url(&self) -> GString
sourcepub fn translator_credits(&self) -> GString
pub fn translator_credits(&self) -> GString
sourcepub fn set_application_icon(&self, application_icon: &str)
pub fn set_application_icon(&self, application_icon: &str)
Sets the name of the application icon for @self.
The icon is displayed at the top of the main page.
§application_icon
the application icon name
sourcepub fn set_application_name(&self, application_name: &str)
pub fn set_application_name(&self, application_name: &str)
Sets the application name for @self.
The name is displayed at the top of the main page.
§application_name
the application name
sourcepub fn set_artists(&self, artists: &[&str])
pub fn set_artists(&self, artists: &[&str])
Sets the list of artists of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
developers
designers
documenters
translator-credits
add_credit_section()
add_acknowledgement_section()
§artists
the list of artists
sourcepub fn set_comments(&self, comments: &str)
pub fn set_comments(&self, comments: &str)
Sets the comments about the application.
Comments will be shown on the Details page, above links.
Unlike [comments
][struct@crate::Gtk::AboutDialog#comments], this string can be long and
detailed. It can also contain links and Pango markup.
§comments
the comments
sourcepub fn set_copyright(&self, copyright: &str)
pub fn set_copyright(&self, copyright: &str)
Sets the copyright information for @self.
This should be a short string of one or two lines, for example:
© 2022 Example
.
The copyright information will be displayed on the Legal page, before the application license.
add_legal_section()
can be used to add copyright
information for the application dependencies or other components.
§copyright
the copyright information
sourcepub fn set_debug_info(&self, debug_info: &str)
pub fn set_debug_info(&self, debug_info: &str)
Sets the debug information for @self.
Debug information will be shown on the Troubleshooting page. It’s intended to be attached to issue reports when reporting issues against the application.
AboutWindow
provides a quick way to save debug information to a file.
When saving, debug-info-filename
would be used as
the suggested filename.
Debug information cannot contain markup or links.
§debug_info
the debug information
sourcepub fn set_debug_info_filename(&self, filename: &str)
pub fn set_debug_info_filename(&self, filename: &str)
Sets the debug information filename for @self.
It will be used as the suggested filename when saving debug information to a file.
See debug-info
.
§filename
the debug info filename
sourcepub fn set_designers(&self, designers: &[&str])
pub fn set_designers(&self, designers: &[&str])
Sets the list of designers of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
developers
artists
documenters
translator-credits
add_credit_section()
add_acknowledgement_section()
§designers
the list of designers
sourcepub fn set_developer_name(&self, developer_name: &str)
pub fn set_developer_name(&self, developer_name: &str)
Sets the developer name for @self.
The developer name is displayed on the main page, under the application name.
If the application is developed by multiple people, the developer name can be
set to values like “AppName team”, “AppName developers” or
“The AppName project”, and the individual contributors can be listed on the
Credits page, with developers
and related properties.
§developer_name
the developer name
sourcepub fn set_developers(&self, developers: &[&str])
pub fn set_developers(&self, developers: &[&str])
Sets the list of developers of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
§developers
the list of developers
sourcepub fn set_documenters(&self, documenters: &[&str])
pub fn set_documenters(&self, documenters: &[&str])
Sets the list of documenters of the application.
It will be displayed on the Credits page.
Each name may contain email addresses and URLs, see the introduction for more details.
See also:
§documenters
the list of documenters
sourcepub fn set_issue_url(&self, issue_url: &str)
pub fn set_issue_url(&self, issue_url: &str)
Sets the issue tracker URL for @self.
The issue tracker link is displayed on the main page.
§issue_url
the issue tracker URL
sourcepub fn set_license(&self, license: &str)
pub fn set_license(&self, license: &str)
Sets the license for @self.
This can be used to set a custom text for the license if it can’t be set via
license-type
.
When set, license-type
will be set to
GTK_LICENSE_CUSTOM
.
The license text will be displayed on the Legal page, below the copyright information.
License text can contain Pango markup and links.
add_legal_section()
can be used to add license information
for the application dependencies or other components.
§license
the license
sourcepub fn set_license_type(&self, license_type: License)
pub fn set_license_type(&self, license_type: License)
Sets the license for @self from a list of known licenses.
If the application’s license is not in the list,
license
can be used instead. The license type will be
automatically set to GTK_LICENSE_CUSTOM
in that case.
If @license_type is GTK_LICENSE_UNKNOWN
, no information will be displayed.
If @license_type is different from GTK_LICENSE_CUSTOM
.
license
will be cleared out.
The license description will be displayed on the Legal page, below the copyright information.
add_legal_section()
can be used to add license information
for the application dependencies or other components.
§license_type
the license type
sourcepub fn set_release_notes(&self, release_notes: &str)
pub fn set_release_notes(&self, release_notes: &str)
Sets the release notes for @self.
Release notes are displayed on the the What’s New page.
Release notes are formatted the same way as AppStream descriptions.
The supported formatting options are:
- Paragraph (
<p>
) - Ordered list (
<ol>
), with list items (<li>
) - Unordered list (
<ul>
), with list items (<li>
)
Within paragraphs and list items, emphasis (<em>
) and inline code
(<code>
) text styles are supported. The emphasis is rendered in italic,
while inline code is shown in a monospaced font.
Any text outside paragraphs or list items is ignored.
Nested lists are not supported.
AboutWindow
displays the version above the release notes. If set, the
release-notes-version
of the property will be used
as the version; otherwise, version
is used.
§release_notes
the release notes
sourcepub fn set_release_notes_version(&self, version: &str)
pub fn set_release_notes_version(&self, version: &str)
Sets the version described by the application’s release notes.
The release notes version is displayed on the What’s New page, above the release notes.
If not set, version
will be used instead.
For example, an application with the current version 2.0.2 might want to keep the release notes from 2.0.0, and set the release notes version accordingly.
See release-notes
.
§version
the release notes version
sourcepub fn set_support_url(&self, support_url: &str)
pub fn set_support_url(&self, support_url: &str)
Sets the URL of the support page for @self.
The support page link is displayed on the main page.
§support_url
the support page URL
sourcepub fn set_translator_credits(&self, translator_credits: &str)
pub fn set_translator_credits(&self, translator_credits: &str)
Sets the translator credits string.
It will be displayed on the Credits page.
This string should be "translator-credits"
or "translator_credits"
and
should be marked as translatable.
The string may contain email addresses and URLs, see the introduction for more details.
See also:
§translator_credits
the translator credits
sourcepub fn set_version(&self, version: &str)
pub fn set_version(&self, version: &str)
Sets the version for @self.
The version is displayed on the main page.
If release-notes-version
is not set, the version will
also be displayed above the release notes on the What’s New page.
§version
the version
sourcepub fn set_website(&self, website: &str)
pub fn set_website(&self, website: &str)
Sets the application website URL for @self.
Website is displayed on the Details page, below comments, or on the main page if the Details page doesn’t have any other content.
Applications can add other links below, see add_link()
.
§website
the website URL
sourcepub fn connect_activate_link<F: Fn(&Self, &str) -> bool + 'static>(
&self,
f: F
) -> SignalHandlerId
pub fn connect_activate_link<F: Fn(&Self, &str) -> bool + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_application_icon_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_application_name_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_artists_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_comments_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_copyright_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_debug_info_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_debug_info_filename_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_designers_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_developer_name_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_developers_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_documenters_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_issue_url_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_license_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_license_type_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_release_notes_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_release_notes_version_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_support_url_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_translator_credits_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_version_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
pub fn connect_website_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
Trait Implementations§
source§impl Clone for AboutWindow
impl Clone for AboutWindow
source§impl Debug for AboutWindow
impl Debug for AboutWindow
source§impl Default for AboutWindow
impl Default for AboutWindow
source§impl HasParamSpec for AboutWindow
impl HasParamSpec for AboutWindow
type ParamSpec = ParamSpecObject
§type SetValue = AboutWindow
type SetValue = AboutWindow
type BuilderFn = fn(_: &str) -> ParamSpecObjectBuilder<'_, AboutWindow>
fn param_spec_builder() -> Self::BuilderFn
source§impl Hash for AboutWindow
impl Hash for AboutWindow
source§impl Ord for AboutWindow
impl Ord for AboutWindow
source§impl ParentClassIs for AboutWindow
impl ParentClassIs for AboutWindow
source§impl<OT: ObjectType> PartialEq<OT> for AboutWindow
impl<OT: ObjectType> PartialEq<OT> for AboutWindow
source§impl<OT: ObjectType> PartialOrd<OT> for AboutWindow
impl<OT: ObjectType> PartialOrd<OT> for AboutWindow
1.0.0 · source§fn le(&self, other: &Rhs) -> bool
fn le(&self, other: &Rhs) -> bool
self
and other
) and is used by the <=
operator. Read moresource§impl StaticType for AboutWindow
impl StaticType for AboutWindow
source§fn static_type() -> Type
fn static_type() -> Type
Self
.impl Eq for AboutWindow
impl IsA<Accessible> for AboutWindow
impl IsA<Buildable> for AboutWindow
impl IsA<ConstraintTarget> for AboutWindow
impl IsA<Native> for AboutWindow
impl IsA<Root> for AboutWindow
impl IsA<ShortcutManager> for AboutWindow
impl IsA<Widget> for AboutWindow
impl IsA<Window> for AboutWindow
impl IsA<Window> for AboutWindow
Auto Trait Implementations§
impl RefUnwindSafe for AboutWindow
impl !Send for AboutWindow
impl !Sync for AboutWindow
impl Unpin for AboutWindow
impl UnwindSafe for AboutWindow
Blanket Implementations§
source§impl<O> AccessibleExt for Owhere
O: IsA<Accessible>,
impl<O> AccessibleExt for Owhere
O: IsA<Accessible>,
fn accessible_role(&self) -> AccessibleRole
fn reset_property(&self, property: AccessibleProperty)
fn reset_relation(&self, relation: AccessibleRelation)
fn reset_state(&self, state: AccessibleState)
fn set_accessible_role(&self, accessible_role: AccessibleRole)
fn connect_accessible_role_notify<F>(&self, f: F) -> SignalHandlerId
source§impl<O> AccessibleExtManual for Owhere
O: IsA<Accessible>,
impl<O> AccessibleExtManual for Owhere
O: IsA<Accessible>,
fn update_property(&self, properties: &[Property<'_>])
fn update_relation(&self, relations: &[Relation<'_>])
fn update_state(&self, states: &[State])
source§impl<O> AdwWindowExt for O
impl<O> AdwWindowExt for O
source§fn add_breakpoint(&self, breakpoint: Breakpoint)
fn add_breakpoint(&self, breakpoint: Breakpoint)
v1_4
only.source§fn current_breakpoint(&self) -> Option<Breakpoint>
fn current_breakpoint(&self) -> Option<Breakpoint>
v1_4
only.source§fn dialogs(&self) -> ListModel
fn dialogs(&self) -> ListModel
v1_5
only.gio::ListModel
that contains the open dialogs of @self. Read moresource§fn visible_dialog(&self) -> Option<Dialog>
fn visible_dialog(&self) -> Option<Dialog>
v1_5
only.source§fn set_content(&self, content: Option<&impl IsA<Widget>>)
fn set_content(&self, content: Option<&impl IsA<Widget>>)
fn connect_content_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
source§fn connect_current_breakpoint_notify<F: Fn(&Self) + 'static>(
&self,
f: F
) -> SignalHandlerId
fn connect_current_breakpoint_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
v1_4
only.source§fn connect_dialogs_notify<F: Fn(&Self) + 'static>(
&self,
f: F
) -> SignalHandlerId
fn connect_dialogs_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
v1_5
only.source§fn connect_visible_dialog_notify<F: Fn(&Self) + 'static>(
&self,
f: F
) -> SignalHandlerId
fn connect_visible_dialog_notify<F: Fn(&Self) + 'static>( &self, f: F ) -> SignalHandlerId
v1_5
only.source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
source§impl<O> BuildableExt for O
impl<O> BuildableExt for O
fn buildable_id(&self) -> Option<GString>
source§impl<T> Cast for Twhere
T: ObjectType,
impl<T> Cast for Twhere
T: ObjectType,
source§fn upcast<T>(self) -> Twhere
T: ObjectType,
Self: IsA<T>,
fn upcast<T>(self) -> Twhere
T: ObjectType,
Self: IsA<T>,
T
. Read moresource§fn upcast_ref<T>(&self) -> &Twhere
T: ObjectType,
Self: IsA<T>,
fn upcast_ref<T>(&self) -> &Twhere
T: ObjectType,
Self: IsA<T>,
T
. Read moresource§fn downcast<T>(self) -> Result<T, Self>where
T: ObjectType,
Self: MayDowncastTo<T>,
fn downcast<T>(self) -> Result<T, Self>where
T: ObjectType,
Self: MayDowncastTo<T>,
T
. Read moresource§fn downcast_ref<T>(&self) -> Option<&T>where
T: ObjectType,
Self: MayDowncastTo<T>,
fn downcast_ref<T>(&self) -> Option<&T>where
T: ObjectType,
Self: MayDowncastTo<T>,
T
. Read moresource§fn dynamic_cast<T>(self) -> Result<T, Self>where
T: ObjectType,
fn dynamic_cast<T>(self) -> Result<T, Self>where
T: ObjectType,
T
. This handles upcasting, downcasting
and casting between interface and interface implementors. All checks are performed at
runtime, while upcast
will do many checks at compile-time already. downcast
will
perform the same checks at runtime as dynamic_cast
, but will also ensure some amount of
compile-time safety. Read moresource§fn dynamic_cast_ref<T>(&self) -> Option<&T>where
T: ObjectType,
fn dynamic_cast_ref<T>(&self) -> Option<&T>where
T: ObjectType,
T
. This handles upcasting, downcasting
and casting between interface and interface implementors. All checks are performed at
runtime, while downcast
and upcast
will do many checks at compile-time already. Read moresource§unsafe fn unsafe_cast<T>(self) -> Twhere
T: ObjectType,
unsafe fn unsafe_cast<T>(self) -> Twhere
T: ObjectType,
T
unconditionally. Read moresource§unsafe fn unsafe_cast_ref<T>(&self) -> &Twhere
T: ObjectType,
unsafe fn unsafe_cast_ref<T>(&self) -> &Twhere
T: ObjectType,
&T
unconditionally. Read moresource§impl<T> FromGlibContainerAsVec<<T as GlibPtrDefault>::GlibType, *const GList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
impl<T> FromGlibContainerAsVec<<T as GlibPtrDefault>::GlibType, *const GList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
source§impl<T> FromGlibContainerAsVec<<T as GlibPtrDefault>::GlibType, *const GPtrArray> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
impl<T> FromGlibContainerAsVec<<T as GlibPtrDefault>::GlibType, *const GPtrArray> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
source§impl<T> FromGlibContainerAsVec<<T as GlibPtrDefault>::GlibType, *const GSList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
impl<T> FromGlibContainerAsVec<<T as GlibPtrDefault>::GlibType, *const GSList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
source§impl<T> FromGlibContainerAsVec<<T as GlibPtrDefault>::GlibType, *mut GList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
impl<T> FromGlibContainerAsVec<<T as GlibPtrDefault>::GlibType, *mut GList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
source§impl<T> FromGlibContainerAsVec<<T as GlibPtrDefault>::GlibType, *mut GPtrArray> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
impl<T> FromGlibContainerAsVec<<T as GlibPtrDefault>::GlibType, *mut GPtrArray> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
source§impl<T> FromGlibContainerAsVec<<T as GlibPtrDefault>::GlibType, *mut GSList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
impl<T> FromGlibContainerAsVec<<T as GlibPtrDefault>::GlibType, *mut GSList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
source§impl<T> FromGlibPtrArrayContainerAsVec<<T as GlibPtrDefault>::GlibType, *const GList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
impl<T> FromGlibPtrArrayContainerAsVec<<T as GlibPtrDefault>::GlibType, *const GList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
unsafe fn from_glib_none_as_vec(ptr: *const GList) -> Vec<T>
unsafe fn from_glib_container_as_vec(_: *const GList) -> Vec<T>
unsafe fn from_glib_full_as_vec(_: *const GList) -> Vec<T>
source§impl<T> FromGlibPtrArrayContainerAsVec<<T as GlibPtrDefault>::GlibType, *const GPtrArray> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
impl<T> FromGlibPtrArrayContainerAsVec<<T as GlibPtrDefault>::GlibType, *const GPtrArray> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
unsafe fn from_glib_none_as_vec(ptr: *const GPtrArray) -> Vec<T>
unsafe fn from_glib_container_as_vec(_: *const GPtrArray) -> Vec<T>
unsafe fn from_glib_full_as_vec(_: *const GPtrArray) -> Vec<T>
source§impl<T> FromGlibPtrArrayContainerAsVec<<T as GlibPtrDefault>::GlibType, *const GSList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
impl<T> FromGlibPtrArrayContainerAsVec<<T as GlibPtrDefault>::GlibType, *const GSList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
unsafe fn from_glib_none_as_vec(ptr: *const GSList) -> Vec<T>
unsafe fn from_glib_container_as_vec(_: *const GSList) -> Vec<T>
unsafe fn from_glib_full_as_vec(_: *const GSList) -> Vec<T>
source§impl<T> FromGlibPtrArrayContainerAsVec<<T as GlibPtrDefault>::GlibType, *mut GList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
impl<T> FromGlibPtrArrayContainerAsVec<<T as GlibPtrDefault>::GlibType, *mut GList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
unsafe fn from_glib_none_as_vec(ptr: *mut GList) -> Vec<T>
unsafe fn from_glib_container_as_vec(ptr: *mut GList) -> Vec<T>
unsafe fn from_glib_full_as_vec(ptr: *mut GList) -> Vec<T>
source§impl<T> FromGlibPtrArrayContainerAsVec<<T as GlibPtrDefault>::GlibType, *mut GPtrArray> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
impl<T> FromGlibPtrArrayContainerAsVec<<T as GlibPtrDefault>::GlibType, *mut GPtrArray> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
unsafe fn from_glib_none_as_vec(ptr: *mut GPtrArray) -> Vec<T>
unsafe fn from_glib_container_as_vec(ptr: *mut GPtrArray) -> Vec<T>
unsafe fn from_glib_full_as_vec(ptr: *mut GPtrArray) -> Vec<T>
source§impl<T> FromGlibPtrArrayContainerAsVec<<T as GlibPtrDefault>::GlibType, *mut GSList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
impl<T> FromGlibPtrArrayContainerAsVec<<T as GlibPtrDefault>::GlibType, *mut GSList> for Twhere
T: GlibPtrDefault + FromGlibPtrNone<<T as GlibPtrDefault>::GlibType> + FromGlibPtrFull<<T as GlibPtrDefault>::GlibType>,
unsafe fn from_glib_none_as_vec(ptr: *mut GSList) -> Vec<T>
unsafe fn from_glib_container_as_vec(ptr: *mut GSList) -> Vec<T>
unsafe fn from_glib_full_as_vec(ptr: *mut GSList) -> Vec<T>
source§impl<O> GObjectPropertyExpressionExt for O
impl<O> GObjectPropertyExpressionExt for O
source§fn property_expression(&self, property_name: &str) -> PropertyExpression
fn property_expression(&self, property_name: &str) -> PropertyExpression
source§fn property_expression_weak(&self, property_name: &str) -> PropertyExpression
fn property_expression_weak(&self, property_name: &str) -> PropertyExpression
source§fn this_expression(property_name: &str) -> PropertyExpression
fn this_expression(property_name: &str) -> PropertyExpression
this
object.source§impl<O> GtkWindowExt for O
impl<O> GtkWindowExt for O
fn close(&self)
fn destroy(&self)
fn fullscreen(&self)
fn fullscreen_on_monitor(&self, monitor: &Monitor)
fn application(&self) -> Option<Application>
fn child(&self) -> Option<Widget>
fn is_decorated(&self) -> bool
fn default_size(&self) -> (i32, i32)
fn default_widget(&self) -> Option<Widget>
fn is_deletable(&self) -> bool
fn must_destroy_with_parent(&self) -> bool
fn focus(&self) -> Option<Widget>
fn gets_focus_visible(&self) -> bool
fn group(&self) -> WindowGroup
fn hides_on_close(&self) -> bool
fn icon_name(&self) -> Option<GString>
fn is_mnemonics_visible(&self) -> bool
fn is_modal(&self) -> bool
fn is_resizable(&self) -> bool
fn title(&self) -> Option<GString>
fn titlebar(&self) -> Option<Widget>
fn transient_for(&self) -> Option<Window>
fn has_group(&self) -> bool
fn is_active(&self) -> bool
fn is_fullscreen(&self) -> bool
fn is_maximized(&self) -> bool
fn maximize(&self)
fn minimize(&self)
fn present(&self)
fn present_with_time(&self, timestamp: u32)
fn set_application(&self, application: Option<&impl IsA<Application>>)
fn set_child(&self, child: Option<&impl IsA<Widget>>)
fn set_decorated(&self, setting: bool)
fn set_default_size(&self, width: i32, height: i32)
fn set_default_widget(&self, default_widget: Option<&impl IsA<Widget>>)
fn set_deletable(&self, setting: bool)
fn set_destroy_with_parent(&self, setting: bool)
fn set_display(&self, display: &impl IsA<Display>)
fn set_focus(&self, focus: Option<&impl IsA<Widget>>)
fn set_focus_visible(&self, setting: bool)
fn set_hide_on_close(&self, setting: bool)
fn set_icon_name(&self, name: Option<&str>)
fn set_mnemonics_visible(&self, setting: bool)
fn set_modal(&self, modal: bool)
fn set_resizable(&self, resizable: bool)
fn set_startup_id(&self, startup_id: &str)
fn set_title(&self, title: Option<&str>)
fn set_titlebar(&self, titlebar: Option<&impl IsA<Widget>>)
fn set_transient_for(&self, parent: Option<&impl IsA<Window>>)
fn unfullscreen(&self)
fn unmaximize(&self)
fn unminimize(&self)
fn default_height(&self) -> i32
fn set_default_height(&self, default_height: i32)
fn default_width(&self) -> i32
fn set_default_width(&self, default_width: i32)
fn focus_widget(&self) -> Option<Widget>
fn set_focus_widget<P>(&self, focus_widget: Option<&P>)
fn is_fullscreened(&self) -> bool
fn set_fullscreened(&self, fullscreened: bool)
fn set_maximized(&self, maximized: bool)
fn connect_activate_default<F>(&self, f: F) -> SignalHandlerId
fn emit_activate_default(&self)
fn connect_activate_focus<F>(&self, f: F) -> SignalHandlerId
fn emit_activate_focus(&self)
fn connect_close_request<F>(&self, f: F) -> SignalHandlerId
fn connect_enable_debugging<F>(&self, f: F) -> SignalHandlerId
fn emit_enable_debugging(&self, toggle: bool) -> bool
fn connect_keys_changed<F>(&self, f: F) -> SignalHandlerId
fn connect_application_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_child_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_decorated_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_default_height_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_default_widget_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_default_width_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_deletable_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_destroy_with_parent_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_display_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_focus_visible_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_focus_widget_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_fullscreened_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_hide_on_close_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_icon_name_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_is_active_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_maximized_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_mnemonics_visible_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_modal_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_resizable_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_startup_id_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_title_notify<F>(&self, f: F) -> SignalHandlerId
fn connect_transient_for_notify<F>(&self, f: F) -> SignalHandlerId
source§impl<T> IntoClosureReturnValue for T
impl<T> IntoClosureReturnValue for T
fn into_closure_return_value(self) -> Option<Value>
source§impl<U> IsSubclassableExt for Uwhere
U: IsClass + ParentClassIs,
impl<U> IsSubclassableExt for Uwhere
U: IsClass + ParentClassIs,
fn parent_class_init<T>(class: &mut Class<U>)
fn parent_instance_init<T>(instance: &mut InitializingObject<T>)
source§impl<T> ObjectExt for Twhere
T: ObjectType,
impl<T> ObjectExt for Twhere
T: ObjectType,
source§fn is<U>(&self) -> boolwhere
U: StaticType,
fn is<U>(&self) -> boolwhere
U: StaticType,
true
if the object is an instance of (can be cast to) T
.source§fn object_class(&self) -> &Class<Object>
fn object_class(&self) -> &Class<Object>
ObjectClass
of the object. Read moresource§fn class_of<U>(&self) -> Option<&Class<U>>where
U: IsClass,
fn class_of<U>(&self) -> Option<&Class<U>>where
U: IsClass,
T
. Read moresource§fn interface<U>(&self) -> Option<InterfaceRef<'_, U>>where
U: IsInterface,
fn interface<U>(&self) -> Option<InterfaceRef<'_, U>>where
U: IsInterface,
T
of the object. Read moresource§fn set_property_from_value(&self, property_name: &str, value: &Value)
fn set_property_from_value(&self, property_name: &str, value: &Value)
source§fn set_properties(&self, property_values: &[(&str, &dyn ToValue)])
fn set_properties(&self, property_values: &[(&str, &dyn ToValue)])
source§fn set_properties_from_value(&self, property_values: &[(&str, Value)])
fn set_properties_from_value(&self, property_values: &[(&str, Value)])
source§fn property<V>(&self, property_name: &str) -> Vwhere
V: for<'b> FromValue<'b> + 'static,
fn property<V>(&self, property_name: &str) -> Vwhere
V: for<'b> FromValue<'b> + 'static,
property_name
of the object and cast it to the type V. Read moresource§fn property_value(&self, property_name: &str) -> Value
fn property_value(&self, property_name: &str) -> Value
property_name
of the object. Read moresource§fn property_type(&self, property_name: &str) -> Option<Type>
fn property_type(&self, property_name: &str) -> Option<Type>
property_name
of this object. Read moresource§fn find_property(&self, property_name: &str) -> Option<ParamSpec>
fn find_property(&self, property_name: &str) -> Option<ParamSpec>
ParamSpec
of the property property_name
of this object.source§fn list_properties(&self) -> PtrSlice<ParamSpec>
fn list_properties(&self) -> PtrSlice<ParamSpec>
ParamSpec
of the properties of this object.source§fn freeze_notify(&self) -> PropertyNotificationFreezeGuard
fn freeze_notify(&self) -> PropertyNotificationFreezeGuard
source§unsafe fn set_qdata<QD>(&self, key: Quark, value: QD)where
QD: 'static,
unsafe fn set_qdata<QD>(&self, key: Quark, value: QD)where
QD: 'static,
key
. Read moresource§unsafe fn qdata<QD>(&self, key: Quark) -> Option<NonNull<QD>>where
QD: 'static,
unsafe fn qdata<QD>(&self, key: Quark) -> Option<NonNull<QD>>where
QD: 'static,
key
. Read moresource§unsafe fn steal_qdata<QD>(&self, key: Quark) -> Option<QD>where
QD: 'static,
unsafe fn steal_qdata<QD>(&self, key: Quark) -> Option<QD>where
QD: 'static,
key
. Read moresource§unsafe fn set_data<QD>(&self, key: &str, value: QD)where
QD: 'static,
unsafe fn set_data<QD>(&self, key: &str, value: QD)where
QD: 'static,
key
. Read moresource§unsafe fn data<QD>(&self, key: &str) -> Option<NonNull<QD>>where
QD: 'static,
unsafe fn data<QD>(&self, key: &str) -> Option<NonNull<QD>>where
QD: 'static,
key
. Read moresource§unsafe fn steal_data<QD>(&self, key: &str) -> Option<QD>where
QD: 'static,
unsafe fn steal_data<QD>(&self, key: &str) -> Option<QD>where
QD: 'static,
key
. Read moresource§fn block_signal(&self, handler_id: &SignalHandlerId)
fn block_signal(&self, handler_id: &SignalHandlerId)
source§fn unblock_signal(&self, handler_id: &SignalHandlerId)
fn unblock_signal(&self, handler_id: &SignalHandlerId)
source§fn stop_signal_emission(&self, signal_id: SignalId, detail: Option<Quark>)
fn stop_signal_emission(&self, signal_id: SignalId, detail: Option<Quark>)
source§fn stop_signal_emission_by_name(&self, signal_name: &str)
fn stop_signal_emission_by_name(&self, signal_name: &str)
source§fn connect<F>(
&self,
signal_name: &str,
after: bool,
callback: F
) -> SignalHandlerId
fn connect<F>( &self, signal_name: &str, after: bool, callback: F ) -> SignalHandlerId
signal_name
on this object. Read moresource§fn connect_id<F>(
&self,
signal_id: SignalId,
details: Option<Quark>,
after: bool,
callback: F
) -> SignalHandlerId
fn connect_id<F>( &self, signal_id: SignalId, details: Option<Quark>, after: bool, callback: F ) -> SignalHandlerId
signal_id
on this object. Read moresource§fn connect_local<F>(
&self,
signal_name: &str,
after: bool,
callback: F
) -> SignalHandlerId
fn connect_local<F>( &self, signal_name: &str, after: bool, callback: F ) -> SignalHandlerId
signal_name
on this object. Read moresource§fn connect_local_id<F>(
&self,
signal_id: SignalId,
details: Option<Quark>,
after: bool,
callback: F
) -> SignalHandlerId
fn connect_local_id<F>( &self, signal_id: SignalId, details: Option<Quark>, after: bool, callback: F ) -> SignalHandlerId
signal_id
on this object. Read moresource§unsafe fn connect_unsafe<F>(
&self,
signal_name: &str,
after: bool,
callback: F
) -> SignalHandlerId
unsafe fn connect_unsafe<F>( &self, signal_name: &str, after: bool, callback: F ) -> SignalHandlerId
signal_name
on this object. Read moresource§unsafe fn connect_unsafe_id<F>(
&self,
signal_id: SignalId,
details: Option<Quark>,
after: bool,
callback: F
) -> SignalHandlerId
unsafe fn connect_unsafe_id<F>( &self, signal_id: SignalId, details: Option<Quark>, after: bool, callback: F ) -> SignalHandlerId
signal_id
on this object. Read moresource§fn connect_closure(
&self,
signal_name: &str,
after: bool,
closure: RustClosure
) -> SignalHandlerId
fn connect_closure( &self, signal_name: &str, after: bool, closure: RustClosure ) -> SignalHandlerId
signal_name
on this object. Read moresource§fn connect_closure_id(
&self,
signal_id: SignalId,
details: Option<Quark>,
after: bool,
closure: RustClosure
) -> SignalHandlerId
fn connect_closure_id( &self, signal_id: SignalId, details: Option<Quark>, after: bool, closure: RustClosure ) -> SignalHandlerId
signal_id
on this object. Read moresource§fn watch_closure(&self, closure: &impl AsRef<Closure>)
fn watch_closure(&self, closure: &impl AsRef<Closure>)
closure
to the lifetime of the object. When
the object’s reference count drops to zero, the closure will be
invalidated. An invalidated closure will ignore any calls to
invoke_with_values
, or
invoke
when using Rust closures.source§fn emit<R>(&self, signal_id: SignalId, args: &[&dyn ToValue]) -> Rwhere
R: TryFromClosureReturnValue,
fn emit<R>(&self, signal_id: SignalId, args: &[&dyn ToValue]) -> Rwhere
R: TryFromClosureReturnValue,
source§fn emit_with_values(&self, signal_id: SignalId, args: &[Value]) -> Option<Value>
fn emit_with_values(&self, signal_id: SignalId, args: &[Value]) -> Option<Value>
Self::emit
but takes Value
for the arguments.source§fn emit_by_name<R>(&self, signal_name: &str, args: &[&dyn ToValue]) -> Rwhere
R: TryFromClosureReturnValue,
fn emit_by_name<R>(&self, signal_name: &str, args: &[&dyn ToValue]) -> Rwhere
R: TryFromClosureReturnValue,
source§fn emit_by_name_with_values(
&self,
signal_name: &str,
args: &[Value]
) -> Option<Value>
fn emit_by_name_with_values( &self, signal_name: &str, args: &[Value] ) -> Option<Value>
source§fn emit_by_name_with_details<R>(
&self,
signal_name: &str,
details: Quark,
args: &[&dyn ToValue]
) -> Rwhere
R: TryFromClosureReturnValue,
fn emit_by_name_with_details<R>(
&self,
signal_name: &str,
details: Quark,
args: &[&dyn ToValue]
) -> Rwhere
R: TryFromClosureReturnValue,
source§fn emit_by_name_with_details_and_values(
&self,
signal_name: &str,
details: Quark,
args: &[Value]
) -> Option<Value>
fn emit_by_name_with_details_and_values( &self, signal_name: &str, details: Quark, args: &[Value] ) -> Option<Value>
source§fn emit_with_details<R>(
&self,
signal_id: SignalId,
details: Quark,
args: &[&dyn ToValue]
) -> Rwhere
R: TryFromClosureReturnValue,
fn emit_with_details<R>(
&self,
signal_id: SignalId,
details: Quark,
args: &[&dyn ToValue]
) -> Rwhere
R: TryFromClosureReturnValue,
source§fn emit_with_details_and_values(
&self,
signal_id: SignalId,
details: Quark,
args: &[Value]
) -> Option<Value>
fn emit_with_details_and_values( &self, signal_id: SignalId, details: Quark, args: &[Value] ) -> Option<Value>
source§fn disconnect(&self, handler_id: SignalHandlerId)
fn disconnect(&self, handler_id: SignalHandlerId)
source§fn connect_notify<F>(&self, name: Option<&str>, f: F) -> SignalHandlerId
fn connect_notify<F>(&self, name: Option<&str>, f: F) -> SignalHandlerId
notify
signal of the object. Read moresource§fn connect_notify_local<F>(&self, name: Option<&str>, f: F) -> SignalHandlerId
fn connect_notify_local<F>(&self, name: Option<&str>, f: F) -> SignalHandlerId
notify
signal of the object. Read moresource§unsafe fn connect_notify_unsafe<F>(
&self,
name: Option<&str>,
f: F
) -> SignalHandlerId
unsafe fn connect_notify_unsafe<F>( &self, name: Option<&str>, f: F ) -> SignalHandlerId
notify
signal of the object. Read more